Chat
Use the Chat
components to create a messaging interface.
<script setup lang="ts">
const messages = [
{
text: 'It\'s over Anakin, I have the high ground.',
align: 'start',
},
{
text: 'You underestimate my power!',
align: 'end'
},
]
</script>
<template>
<Chat
v-for="message in messages" :key="message.text"
:align="message.align"
>
<ChatBubble>
{{ message.text }}
</ChatBubble>
</Chat>
</template>
Components
The Chat
components include the following:
Chat
The Chat
component wraps the below components. Its styles and behavior can be customized with following props:
Prop | Type | Description |
---|---|---|
pre | boolean | pre-wraps the whitespace, so special characters like \n will work |
align | string | sets alignment to either of the below prop names |
   start | boolean | sets alignment to start |
   end | boolean | sets alignment to end |
ChatBubble
The ChatBubble
contains the text of the message. Its styles can be customized with following props:
Prop | Type | Description |
---|---|---|
color | string | Sets the chat bubble's color to one of the below prop names. |
   neutral | boolean | Sets the color to neutral. |
   primary | boolean | Sets the color to primary. |
   secondary | boolean | Sets the color to secondary. |
   accent | boolean | Sets the color to accent. |
   info | boolean | Sets the color to info. |
   success | boolean | Sets the color to success. |
   warning | boolean | Sets the color to warning. |
   error | boolean | Sets the color to error. |
ChatHeader
The ChatHeader
allows placing text above the ChatBubble
.
ChatFooter
The ChatFooter
allows placing text below the ChatBubble
.
ChatImage
The ChatImage
component shows the user's image. It can be used the same as the Avatar component.
Pre
Use the pre
prop to honor newlines characters (\n
). This example is the same as above, but honors the
newline character in the first message:
<script setup lang="ts">
const messages = [
{
text: 'It\'s over Anakin, \nI have the high ground.',
align: 'start',
},
{
text: 'You underestimate my power!',
align: 'end',
},
]
</script>
<template>
<Chat pre
v-for="message in messages" :key="message.text"
:align="message.align"
>
<ChatBubble>
{{ message.text }}
</ChatBubble>
</Chat>
</template>
With Avatars
Use the ChatImage
component the same as Avatar. Place an image inside and
it will conform to the shape you specify.

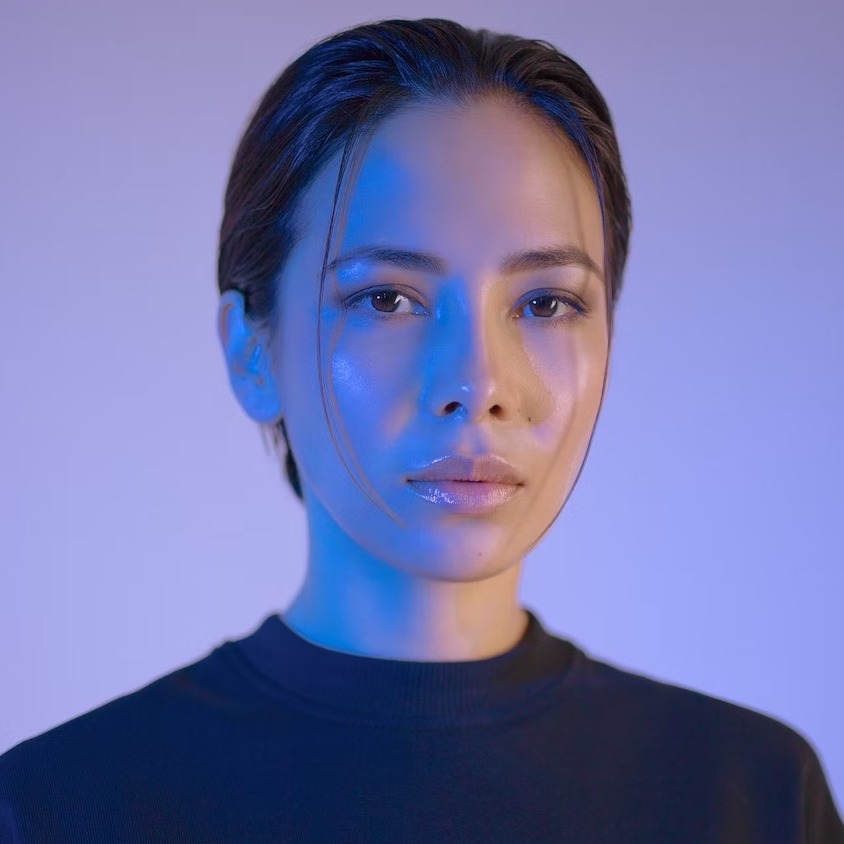
<script setup lang="ts">
const messages = [
{
text: 'It\'s over Anakin, \nI have the high ground.',
align: 'start',
avatar: '/img/avatar-pink.png',
},
{
text: 'You underestimate my power!',
align: 'end',
avatar: '/img/avatar-purple.jpg',
},
]
</script>
<template>
<Chat
v-for="message in messages" :key="message.text"
:align="message.align"
>
<ChatImage circle class="w-12 shrink-0">
<img :src="message.avatar">
</ChatImage>
<ChatBubble>
{{ message.text }}
</ChatBubble>
</Chat>
</template>
Color
Use the color
props to specify color on the ChatBubble
elements.

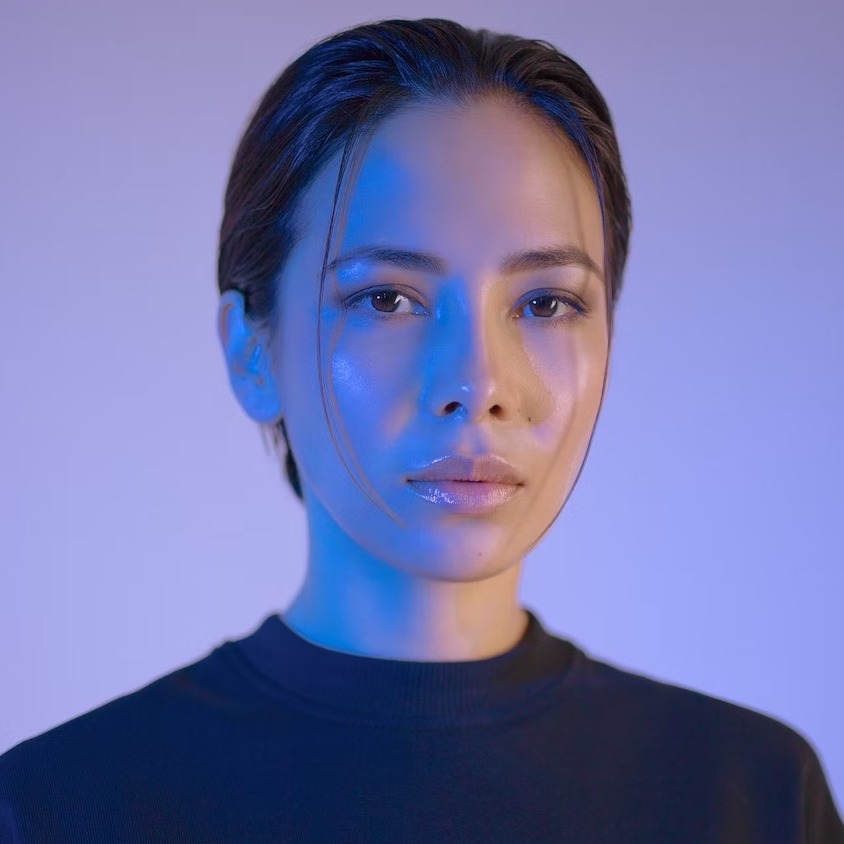
<script setup lang="ts">
const messages = [
{
text: 'It\'s over Anakin, \nI have the high ground.',
align: 'start',
avatar: '/img/avatar-pink.png',
color: 'primary',
},
{
text: 'You underestimate my power!',
align: 'end',
avatar: '/img/avatar-purple.jpg',
color: 'secondary',
},
]
</script>
<template>
<Chat
v-for="message in messages" :key="message.text"
:align="message.align"
>
<ChatImage circle class="w-12 shrink-0">
<img :src="message.avatar">
</ChatImage>
<ChatBubble :color="message.color">
{{ message.text }}
</ChatBubble>
</Chat>
</template>
With Header and Footer
This example makes use of the ChatHeader
and ChatFooter
components.

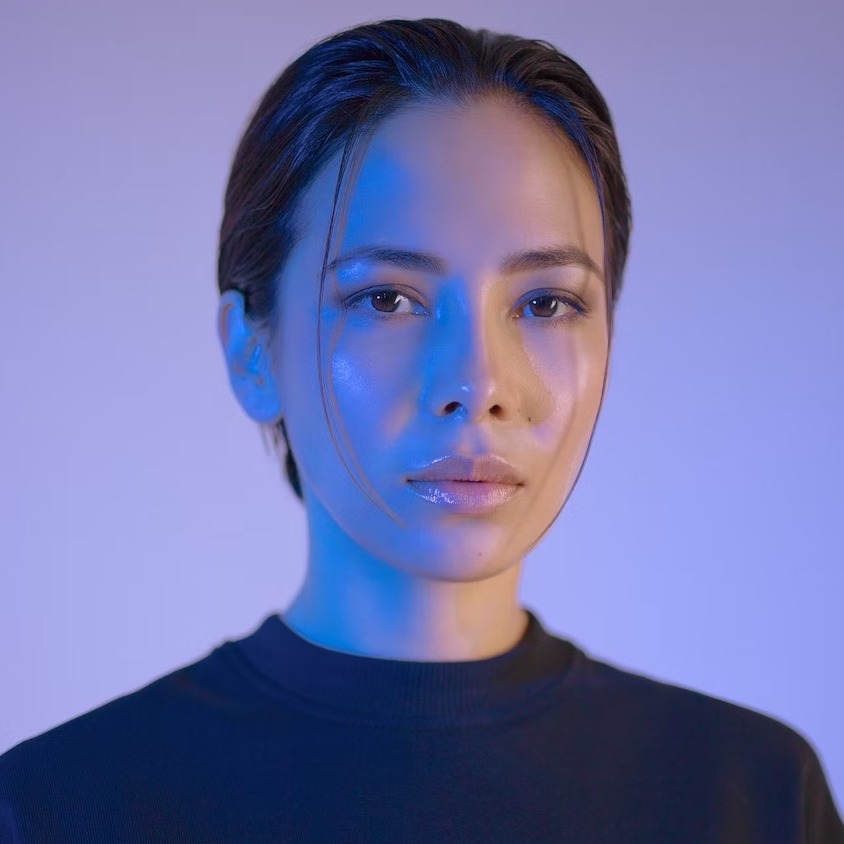
<script setup lang="ts">
const messages = [
{
username: 'Obi-Wan Kenobi',
text: 'You were the chosen one!',
align: 'start',
avatar: '/img/avatar-pink.png',
status: 'Delivered',
time: '2 hours ago',
},
{
username: 'Anakin Skywalker',
text: 'I hate you!',
align: 'end',
avatar: '/img/avatar-purple.jpg',
status: 'Read',
time: '12:46pm',
},
]
</script>
<template>
<Chat
v-for="message in messages" :key="message.text"
:align="message.align"
>
<ChatImage circle class="w-12 shrink-0">
<img :src="message.avatar">
</ChatImage>
<ChatHeader>
{{ message.username }}
</ChatHeader>
<ChatBubble>
{{ message.text }}
</ChatBubble>
<ChatFooter>
{{ message.status }}
<time class="text-xs opacity-50">{{ message.time }}</time>
</ChatFooter>
</Chat>
</template>